Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
Tags
- 정렬
- 시뮬레이션
- 그리디 알고리즘
- 구현
- 자료구조
- 스택
- 백트래킹
- DFS
- c++
- 그래프
- 브루트포스
- VR
- 다익스트라
- 재귀
- 알고리즘
- 투 포인터
- 트리
- BFS
- 백준
- 문자열
- XR Interaction Toolkit
- Team Fortress 2
- 다이나믹 프로그래밍
- 우선순위 큐
- 유니티
- 수학
- ue5
- 유니온 파인드
- 누적 합
- Unreal Engine 5
Archives
- Today
- Total
1일1알
백준 2310번 어드벤처 게임 C++ 본문
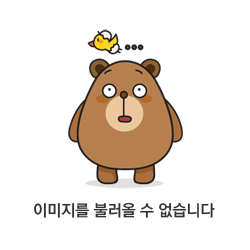
방문표시를 어떤 금액을 가지고 어떤 방을 들어갔는지 정보를 가지고 하면 bfs로 쉽게 풀 수 있다.
#include <iostream>
#include <string>
#include <vector>
#include <math.h>
#include <algorithm>
#include <utility>
#include <stack>
#include <queue>
#include <math.h>
#include <set>
#include <map>
#include <list>
#include <unordered_map>
#include <unordered_set>
#include <iomanip>
#include <limits.h>
using namespace std;
using int64 = long long;
int n;
struct RoomInfo {
char type;
int cost;
vector<int> nextRooms;
};
int main() {
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
while (true) {
cin >> n;
if (n == 0)
break;
vector<vector<bool>> found(n + 1, vector<bool>(501, false));
vector<RoomInfo> RoomInfos(n + 1);
for (int i = 1; i <= n; i++) {
char type;
int cost;
cin >> type >> cost;
vector<int> nextRooms;
while (true) {
int nextRoom;
cin >> nextRoom;
if (nextRoom == 0)
break;
nextRooms.push_back(nextRoom);
}
RoomInfos[i] = { type,cost,nextRooms };
}
queue<pair<int, int>> q;
int startCost = 0;
if (RoomInfos[1].type == 'L') {
startCost = max(startCost, RoomInfos[1].cost);
}
if (RoomInfos[1].type == 'T') {
startCost -= RoomInfos[1].cost;
}
if (startCost >= 0) {
q.push({ 1,startCost });
found[1][startCost] = true;
}
bool ans = false;
while (!q.empty()) {
auto curr = q.front();
q.pop();
if (curr.first == n) {
ans = true;
break;
}
int currCost = curr.second;
for (auto nextRoomIdx : RoomInfos[curr.first].nextRooms) {
int nextCost = currCost;
if (RoomInfos[nextRoomIdx].type == 'L') {
nextCost = max(nextCost, RoomInfos[nextRoomIdx].cost);
}
else if (RoomInfos[nextRoomIdx].type == 'T') {
nextCost -= RoomInfos[nextRoomIdx].cost;
}
if (nextCost < 0) continue;
if (found[nextRoomIdx][nextCost]) continue;
q.push({ nextRoomIdx,nextCost });
found[nextRoomIdx][nextCost] = true;
}
}
if (ans)
cout << "Yes";
else
cout << "No";
cout << "\n";
}
}
'알고리즘' 카테고리의 다른 글
백준 4991번 로봇 청소기 C++ (0) | 2022.07.26 |
---|---|
백준 3197번 백조의 호수 C++ (0) | 2022.07.25 |
백준 16946번 벽 부수고 이동하기 4 C++ (0) | 2022.07.23 |
백준 16724번 피리 부는 사나이 C++ (0) | 2022.07.22 |
백준 2342번 Dance Dance Revolution C++ (0) | 2022.07.21 |